You’ve been there, haven’t you? Pouring over lines of Python code late at night, your eyes glossing over as you stare at a seemingly nonsensical message that’s halted your progress: “ValueError: Columns must be the same length as key”. You’re not alone – even the most seasoned coders have found themselves in this pickle. When this error message flashes on your screen, it’s the Python interpreter’s way of telling you that the lengths of your columns and keys in your data structure aren’t aligning. Maybe you’re working on a pandas DataFrame or perhaps it’s a simple dictionary; regardless, this hiccup is likely disrupting your workflow. But don’t worry, this isn’t an insurmountable hurdle.
Think of it as an opportunity, a problem-solving adventure in the world of coding. It’s all part of the thrilling journey of being a programmer. Here’s the good news: you have the power to fix this! So buckle up, put on your problem-solving hat, and get ready. Here’s your roadmap to resolving “ValueError: Columns must be the same length as key”.
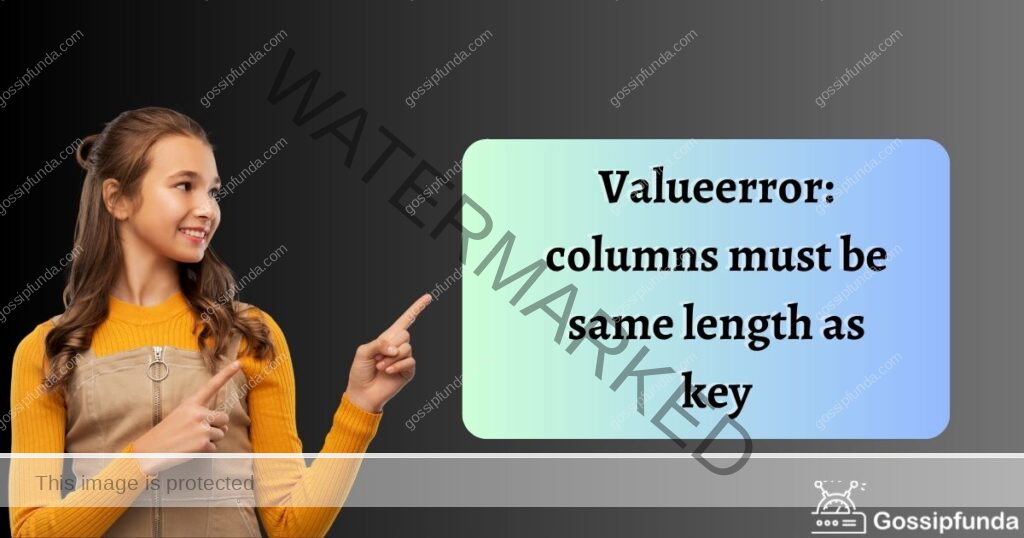
Also read: Awakened Poe trade error code 1020
Causes for Valueerror: columns must be same length as key
Mismatch in DataFrame Column Lengths
One of the primary reasons you might encounter the “ValueError: Columns must be the same length as key” message is due to mismatched DataFrame column lengths in your Python code. DataFrame is a two-dimensional labeled data structure in pandas. You could be dealing with a DataFrame where the columns do not align with the keys. Imagine working on a table where the number of chairs doesn’t match the number of guests. Uncomfortable, right? In the world of coding, such mismatches cause errors.
Remember, DataFrame is like an Excel spreadsheet. Each column should have the same number of entries. Suppose you’ve got three columns – ‘Names’, ‘Ages’, and ‘Professions’ – and you try to insert four items into ‘Names’ and only three into ‘Ages’ and ‘Professions’. Python will halt, it can’t handle this mismatch. Keeping a keen eye on the length of your entries when constructing your DataFrames can save you from the dreaded ValueError.
Inconsistent Dictionary Key-Value Pairs
Another culprit behind the “ValueError: Columns must be the same length as key” is an imbalance in the number of keys and values in a Python dictionary. Think of a dictionary as a simple mapping between a set of keys and a set of values. Picture a locker room where each key should open a specific locker. Now, what happens when you’ve got extra keys or extra lockers? It creates confusion, and in Python, it throws a ValueError.
So, when you’re dealing with dictionaries, ensure each key has a corresponding value. This consistency will keep your code running smoothly, and more importantly, error-free.
Mishandling of MultiIndex DataFrame
Finally, the error could be a result of mishandling a MultiIndex DataFrame. These are DataFrames with multiple levels of indexing on one or both axes. Imagine you have a DataFrame of students, with indices by school and grade. If you attempt to manipulate this DataFrame incorrectly, such as adding a column without specifying the appropriate level, Python will stop you in your tracks.
The key here is to understand the structure of your MultiIndex DataFrame and carefully plan your operations to match that structure. It’s about ensuring harmony and balance in your data to keep Python content and your coding journey on track.
Incorrect Use of the Merge Function
When you’re merging two DataFrames, you can fall into the trap of the “ValueError: Columns must be the same length as key” if the merging isn’t implemented correctly. Merging is a powerful tool in pandas, allowing you to join two data sets together. But if the keys you’re merging on don’t align perfectly in both data sets, Python is going to flash that error message. So next time you’re using the merge function, double-check your keys. They are the bridge between your data sets, and they need to be a perfect match.
Faulty DataFrame Concatenation
Concatenation, or appending rows from multiple DataFrames into one, is another common source of this error. It’s like stacking Lego blocks – all blocks need to fit perfectly. Suppose you’re trying to concatenate DataFrames with differing numbers of columns or inconsistent indices. Python won’t let you off the hook, and it’s going to call you out with the ValueError. Remember, concatenation requires alignment. It’s crucial to ensure your DataFrames line up nicely before you attempt to concatenate them.
Improper Use of the GroupBy Method
The use of the GroupBy method can also result in the ValueError. GroupBy is an invaluable tool for segmenting a DataFrame into groups to perform actions on. However, if you’re attempting to apply a function that changes the length of the groups, you’re going to run into trouble. When using GroupBy, always ensure that any function you’re applying maintains the original length of your groups.
Misuse of the Pivot Method
The pivot method in pandas is another potential trap. This function reshapes your DataFrame, turning columns into headers. But if you pivot without specifying the right parameters or your DataFrame has duplicate entries, you could be in for a surprise. Python is going to throw a ValueError if it sees anything out of place. Always be cautious and specific when using the pivot method, and ensure your data is properly structured for the transformation.
Faulty Reshaping with the Pivot_table Method
When you’re dealing with the Python pandas library, one vital function that could potentially lead to the dreaded “ValueError: Columns must be the same length as key” is the pivot_table method. This function, which is often employed to reshape your DataFrame for analysis purposes, transforms your columns into new headers. It’s a powerful tool that can help you summarize and visualize your data more effectively. However, as with all good things, it can create trouble if not used with caution.
What could go wrong, you ask? Suppose you have a DataFrame that contains duplicate entries – instances where the same set of values occur more than once across your columns. Now imagine trying to pivot this DataFrame. Python is like a perfectionist artist that wants each part of its work – your DataFrame – to fit perfectly in its place. If it sees these duplicate entries during the pivot, it won’t know where to place them. This confusion leads Python to halt the operation and throw a ValueError, informing you that your data isn’t structured correctly for a pivot transformation.
To avoid this, it’s essential to ensure your data is free from duplicates before executing a pivot operation. Additionally, paying close attention to the parameters you pass into the pivot_table function can make a world of difference. It’s a little like cooking – the right ingredients, in the right amounts, make the perfect dish. In this case, your dish is a well-structured DataFrame, and your ingredients are the correct parameters and data.
Inappropriate Use of the Join Method
Another pitfall that could lead to our error is the join method, a function used to combine DataFrames based on a related column, or “key”. When you attempt to join two DataFrames that don’t have matching keys, it’s like trying to solve a puzzle with pieces from different sets. Your DataFrame keys should correspond perfectly to avoid the ValueError.
Furthermore, if one DataFrame contains more instances of a key than the other, Python will stop you in your tracks. For example, suppose you have two DataFrames – one detailing students’ names and another with their respective test scores. If one DataFrame contains multiple entries for the same student, while the other only has one, Python will throw a ValueError when you attempt to join.
Therefore, you should ensure your data is consistently structured and that keys are unique, or, if duplicated, duplicated across all DataFrames. Just like in a well-oiled machine, all parts need to fit together perfectly. When all your DataFrame “parts” align, Python will happily execute your join operation, bringing you one step closer to your data analysis goal.
Errors with the Reshape Method
Lastly, the reshape method, specifically used in the numpy library, is a common error spot. Reshaping allows us to change the number of dimensions and layout of an array without changing its data. It’s like a blacksmith forging a new shape from a piece of metal, except you’re working with arrays. However, if you’re not careful with the new dimensions you’re specifying, you can easily walk into the ValueError.
Here’s an example: suppose you have a one-dimensional array with 12 elements, and you want to reshape it into a two-dimensional one. If you specify dimensions that cannot be filled by exactly 12 elements – say, a 5×3 array which would require 15 elements – Python can’t perform the operation. The ValueError it throws is its way of saying, “Hey, I can’t fit these 12 elements into the shape you want.”
To avoid this, always ensure the total size of the new array matches the size of the original one. For instance, reshaping a 12-element array into a 4×3 or 2×6 array would work perfectly. Remember, reshaping is about rearranging, not adding or subtracting. Keeping this in mind will ensure you use the reshape method effectively and error-free.
How to fix Valueerror: columns must be same length as key
Fix 1. Step-By-Step Indexing Evaluation
Step 1: Start by identifying the issue. Before you make any changes to your code, take a moment to understand the problem. Inspect your DataFrame or dictionary structure for any inconsistencies. You’re looking for mismatches in lengths that might be causing the ValueError.
Step 2: Next, evaluate your index structure. If the index doesn’t align with your data, it could be the root of the problem. Look for any disparities between the index and the data in terms of length and structure.
Step 3: Adjust the index if needed. If you’ve identified an inconsistency, take action to align your index with your data. This adjustment could be as simple as resetting your index or restructuring it entirely.
Step 4: Test your code again. After making the necessary adjustments, run your code to see if the issue persists. If your index was the problem, your code should now execute without the ValueError.
Fix 2. Thorough Code Adjustments
Step 1: Identify the root of the issue. Begin by pinpointing the parts of your code that could be causing the error. You’re on the lookout for any data structure where the length of keys doesn’t match the length of values.
Step 2: Implement changes in your code structure. Once you’ve identified the problem, make the necessary changes to your code. It might involve adjusting the way you’re inputting data or restructuring your DataFrame or dictionary.
Step 3: Test your modified code. After implementing the changes, test your code again. If you’ve correctly adjusted the data input, your code should now execute without the error.
Fix 3. Detailed Data Preprocessing
Step 1: Start by examining your data set. Look for any duplicates that could be causing the ValueError when executing operations like pivot, join, or merge.
Step 2: Implement data cleaning procedures. If you find duplicates, clean your data set by dropping these instances. Python’s pandas library provides functions like drop_duplicates() for this purpose.
Step 3: Verify the cleaned data set. After cleaning your data, check if it’s now structured correctly for your intended operation.
Step 4: Test your operation again. Execute your operation on the cleaned data set. If the duplicates were the problem, your operation should now complete without throwing the ValueError.
Fix 4. Utilizing the ‘ignore_index’ Parameter
Step 1: Analyze the context of your error. If the “ValueError: Columns must be same length as key” error arises while you’re concatenating or appending DataFrames, there’s a chance that the problem lies in mismatched indices.
Step 2: Implement the ‘ignore_index’ parameter. In the concat() or append() function, set ignore_index=True. This action tells pandas to create a new index for your resultant DataFrame, disregarding the existing index.
Step 3: Verify the operation. After incorporating ‘ignore_index’, take a look at your DataFrame. The indices should now range from 0 to the length of your DataFrame minus one.
Step 4: Run your code again. If the indices were the culprit, your code should execute without errors now.
Fix 5. Turning to External Libraries
Step 1: Consider using an external library. If none of the previous solutions have worked, it might be time to consider using a library like NumPy, known for its flexible data handling.
Step 2: Implement NumPy’s array functionality. Transform your data structure into a NumPy array. These structures allow more flexibility in data manipulation and might provide an alternative way to execute your operation.
Step 3: Verify your NumPy array. Once your data is in array format, inspect it to ensure it’s structured correctly.
Step 4: Execute your operation with the NumPy array. If the error was due to rigid data structure limitations, the flexibility of NumPy arrays should allow your operation to execute successfully.
Fix 6. Reconfiguring Data Input
Step 1: Reevaluate your data input. Take a close look at the data you’re feeding into your DataFrame or dictionary. Are there mismatches in lengths of keys and values?
Step 2: Restructure your data input. If you spot discrepancies, restructure your data input to ensure there’s a corresponding value for each key.
Step 3: Verify your restructured data input. After restructuring, confirm that your data input is now consistent.
Step 4: Reattempt your operation. With the corrected data input, try your operation again. It should now proceed without any errors.
Fix 7. Exploring List Comprehensions
Step 1: Identify potential simplifications in your code. If your code is overly complex, it might be causing the ValueError. Can your operation be simplified using a list comprehension?
Step 2: Implement a list comprehension. Transform complex operations into a more straightforward list comprehension. These structures can often streamline your code, avoiding errors caused by overcomplicated procedures.
Step 3: Test the list comprehension. Run your code with the newly implemented list comprehension. If complexity was the problem, your code should now execute error-free.
Fix 8. Checking DataFrame Consistency
Step 1: Inspect your DataFrame for inconsistencies. If certain operations are throwing the ValueError, there might be inconsistencies in your DataFrame’s structure.
Step 2: Ensure data types are uniform. Make sure that all values under a specific column are of the same data type. Use the astype() function if needed to convert data types.
Step 3: Check for missing values. Use the isnull() function to find any missing values in your DataFrame. If you find any, decide on a suitable method to handle them – dropping, filling, or ignoring.
Step 4: Try your operation again. After ensuring the consistency of your DataFrame, your operation should proceed without throwing the ValueError.
Fix 9. Re-evaluating Merge Operations
Step 1: Assess your merging operation. If you’re using pandas merge function and encountering the “ValueError: Columns must be same length as key”, you might be dealing with mismatched keys in the DataFrames you’re trying to merge.
Step 2: Inspect the keys you’re merging on. Check to see if these keys are present in both DataFrames and whether they have corresponding values in each.
Step 3: Adjust your merge operation. If you find mismatches, you may need to adjust your merge operation or the DataFrames themselves to ensure compatibility.
Step 4: Reattempt the merge. With your modified merge operation or DataFrames, try the merge again. The operation should now run smoothly without triggering the ValueError.
Fix 10. Changing the way of Data Slicing
Step 1: Look at how you’re slicing your data. If you’re encountering the ValueError during a slicing operation, the way you’re slicing your data could be the problem.
Step 2: Reconsider your slicing method. Are you trying to slice more data than actually exists in your DataFrame or array? If so, you’ll need to adjust your slicing to fit within the bounds of your data.
Step 3: Implement the adjusted slicing operation. Change your slicing operation to ensure it’s within the bounds of your data.
Step 4: Test your code again. With the adjusted slicing operation, try running your code. If out-of-bound slicing was the issue, your code should now execute without any errors.
Fix 11. Checking for Unsorted Indices
Step 1: Inspect your indices. If you’re dealing with unsorted indices, certain pandas operations could trigger the ValueError.
Step 2: Sort your indices. Use pandas sort_index() function to ensure your DataFrame’s indices are in order.
Step 3: Verify the sorted DataFrame. After sorting, check that your DataFrame’s indices are now in the correct order.
Step 4: Run your code again. With sorted indices, your code should execute successfully, sidestepping the ValueError.
Fix 12. Using the ‘drop’ Parameter
Step 1: Consider dropping the problematic column. If a specific column in your DataFrame is causing the ValueError and isn’t vital for your operation, consider dropping it.
Step 2: Implement the ‘drop’ function. In pandas, use the drop() function, passing the name of the problematic column.
Step 3: Check your DataFrame after dropping the column. Ensure the problematic column has been removed successfully.
Step 4: Test your operation again. With the problematic column removed, your operation should now execute without the ValueError.
Fix 13. Transforming the DataFrame to a Series
Step 1: Evaluate your operation’s needs. If you’re working with a single-column DataFrame and encountering the ValueError, it might be more effective to operate on a pandas Series instead.
Step 2: Transform your DataFrame to a Series. Use the squeeze() function in pandas to transform your single-column DataFrame into a Series.
Step 3: Verify the transformation. Ensure your DataFrame has been successfully transformed into a Series.
Step 4: Reattempt your operation on the Series. If the DataFrame structure was the issue, your operation should now proceed smoothly on the Series.
Conclusion
So, you’ve dived deep into the abyss of Python’s “ValueError: columns must be same length as key.” It’s a common issue, sure, but it’s a fixable one. It doesn’t matter if the trouble started with mismatched data lengths, duplicate values, or the unforgiving reality of incorrect data types. Or perhaps you’re wrestling with misaligned indices, unsorted data, or complexities of code. The key is, you’re not alone. Thousands of coders have met this challenge, just like you, and found their way past it. The beauty of Python, and coding in general, lies in its capacity for problem-solving. You’ve now got an arsenal of strategies, fixes, and approaches that can turn that dreaded “ValueError” into a moment of triumph. Remember, every error is a stepping stone on the journey of programming. Happy coding!
FAQs
This error typically appears when the lengths of your keys and values don’t match in a data structure.
Methods include checking your DataFrame’s structure, cleaning duplicates, ensuring uniform data types, and managing your DataFrame’s indices.
Yes, you can avoid it by ensuring that the keys you’re merging on are present and compatible in both DataFrames.
Ensure your slicing operation is within the bounds of your data to avoid this error.
Transforming your single-column DataFrame to a Series using the squeeze() function can help avoid this error.

I am Shatakshi Mishra.
Currently pursuing my bachelor degree in computer science and engineering from Lovely Professional University, Punjab.
Knowledge needs to be channelled and creative writing is the key to it. Blogging appears to be the best way for sharing my resources and knowledge to the community outside there, helping them and in return learning too from them.