In the world of web development, encountering a JSON parse error marked by an “unexpected token” can halt your project in its tracks. This error is a common roadblock that both novice and seasoned developers may face when dealing with JSON data. Understanding what this error means and how to troubleshoot it is crucial for maintaining the efficiency of your code and ensuring a seamless user experience.
Reasons behind json parse error unexpected token
When developers encounter a JSON parse error with an unexpected token, it’s often a sign that something is amiss in the data structure. The JSON format is unforgiving; a single misplaced comma or incorrectly placed quote can cause the entire data structure to become unreadable. Here are some key reasons behind these errors:
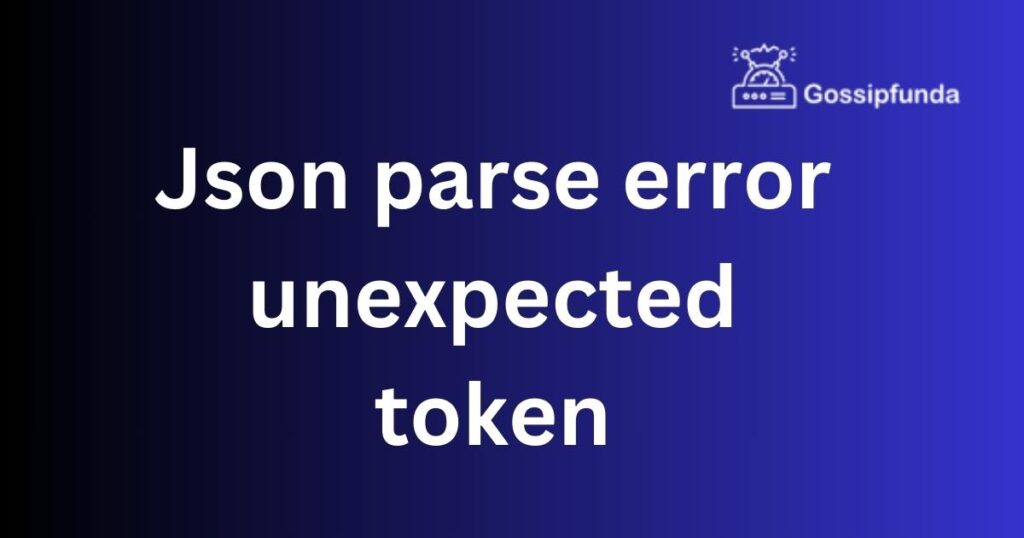
- Improper Syntax: The most common culprit is incorrect syntax. JSON requires specific punctuation and structure. Every string must be enclosed in double quotes, objects wrapped in curly braces, and arrays in square brackets. A deviation from this can lead to unexpected token errors.
- Data Types: JSON supports specific data types: numbers, strings, boolean, arrays, objects, and null. If a value doesn’t conform to these types, or if a number comes across as a string, an unexpected token error might be thrown.
- Comma Errors: Whether it’s a missing comma between elements or an extra comma after the last element in an array or object, comma misuse can throw off the parser. JSON does not tolerate trailing commas.
- Quotation Marks: Unlike some other scripting languages, JSON specifies the use of double quotes for strings and property names. Single quotes or the absence of quotes where expected can result in parsing errors.
- Unescaped Characters: Within a string, certain characters must be escaped with a backslash (). These include quotes within strings, backslashes themselves, and control characters like newlines (\n) and tabs (\t).
- Encoding Issues: Sometimes, the file encoding can cause issues. JSON is typically encoded in UTF-8. If the encoding is mismatched, the parser might read the wrong token, resulting in an error.
- Unexpected Data: Unexpected additional data at the end of a JSON string can also cause parsing to fail. This often happens when concatenating JSON strings or when extra data is inadvertently included.
- Infinite Recursion: If the JSON object has deeply nested objects or arrays, it could potentially exceed the parser’s maximum recursion depth, leading to an unexpected token error.
- File Corruption: At times, the issue could be due to corrupt files or badly formed data from the start. If a JSON file is edited with a program that doesn’t preserve its formatting, it could introduce errors.
- BOM Character: The Byte Order Mark (BOM) is a Unicode character that sometimes precedes the text in UTF-8 files. Not all JSON parsers handle the BOM correctly, and it may lead to an unexpected token at the beginning of your JSON text.
How to fix json parse error unexpected token?
Fix 1: Correcting Syntax Errors
Syntax errors are the primary culprits behind JSON parse error unexpected token issues. The JSON format is extremely particular about syntax. To resolve these errors, follow these detailed steps:
- Step 1: Validate Your JSON: The first step is to check your JSON file against a JSON validator. There are many online tools available that can help you identify where your JSON syntax is incorrect. Just copy and paste your JSON data into the validator, and it will highlight any syntax issues.
- Step 2: Double-Check Your Brackets: Ensure that all your curly braces {} and square brackets [] are correctly paired. Every opening brace or bracket should have a corresponding closing brace or bracket. Unmatched pairs are a common source of syntax errors.
- Step 3: Verify Your Quotes: Check that all your strings and property names are enclosed in double quotes. If you’ve mistakenly used single quotes or have omitted quotes altogether, this will result in a syntax error. Replace any single quotes with double quotes and ensure that all strings are properly quoted.
- Step 4: Comma Placement: Look for missing or extra commas. In a JSON object or array, each key-value pair or element should be followed by a comma, except for the last one. Remove any trailing commas after the last element, and add missing commas where necessary.
- Step 5: Correct Data Types: Ensure that the data types are as expected. Numbers should not be enclosed in quotes, booleans should be written as true or false without quotes, and null should also be without quotes. Correct any mismatches in your JSON file.
- Step 6: Escape Special Characters: For any special characters in your strings, like backslashes or quotes, make sure they are properly escaped with a backslash (\). For example, to include a double quote within a string, it should be written as \”.
- Step 7: Save and Revalidate: After making the necessary corrections, save your file and run it through the JSON validator again to ensure that all syntax errors have been resolved. If the validator gives you the green light, the syntax errors have been fixed, and you should be able to parse your JSON without encountering the unexpected token error.
- Step 8: Test in Your Environment: Finally, it’s time to test the JSON in your development environment. Use the same JSON parsing method that previously triggered the error and see if the issue persists. If your syntax was the problem, your JSON should now parse without any issues.
- Step 9: Use Syntax Highlighting Editors: To prevent future syntax errors, consider using a code editor with JSON syntax highlighting. These editors can help you spot errors as you type, reducing the likelihood of syntax-related unexpected token errors.
By systematically following these steps, developers can efficiently resolve syntax errors, thereby eliminating the JSON parse error unexpected token from their development woes.
Don’t miss: Error Code 4-402 | SLING TV help
Fix 2: Handling Data Types and Structure
JSON is sensitive not just to syntax but also to the proper structure and data types. If your values are not in the correct format, it can result in JSON parse error unexpected token issues. Here’s how you can address these errors by carefully handling data types and structures:
- Step 1: Assess Data Types: Ensure all your data types are correctly used. Numbers should be unquoted, booleans should be in lowercase (true or false), and null values should be represented by the keyword null without quotes. Strings should always be in double quotes.
- Step 2: Inspect JSON Structure: Check your JSON file for structural accuracy. An array must be in square brackets and an object in curly braces. Make sure the structure you are using accurately reflects the data you intend to represent—objects for named properties and arrays for ordered collections.
- Step 3: Array and Object Integrity: Within arrays and objects, maintain integrity by ensuring that they are homogeneous if necessary. Arrays should typically hold elements of the same data type unless your use case specifically calls for a mixed-type array. Objects should not have nested properties that conflict with top-level properties.
- Step 4: Nested Objects: If you’re working with nested objects or arrays, confirm that they’re correctly formatted. Each nested object or array should be well-contained within its parent object or array, following all the same rules regarding commas, quotation marks, and data types.
- Step 5: Remove Extra Data: Oftentimes, developers accidentally leave extra data at the end of a JSON object or array that can cause parsing to fail. After the closing bracket or brace, there should be no additional characters or tokens, except for a comma if it’s part of a larger structure.
- Step 6: Cross-check with a Code Example: If you’re unsure about the structure, compare your JSON to a verified example of correctly structured JSON that has similar data. This can often help you spot structural discrepancies in your own code.
- Step 7: Use a Linting Tool: A linting tool can help enforce standards and catch structural issues in your JSON file. Most Integrated Development Environments (IDEs) have plugins or built-in support for JSON linting.
- Step 8: Test Your Fixes: After making adjustments, test the JSON data again in your environment to see if the issue is resolved. It’s a good practice to test your JSON in a development environment where you can safely see if the unexpected token error persists.
- Step 9: Consistency in Key Naming: Make sure that your object keys are consistent throughout your JSON. Inconsistent keys or typo in key names can cause unexpected behavior when the data is parsed into objects in your programming environment.
By following these steps, developers can ensure that their JSON data is not only syntactically correct but also structurally sound and appropriately typed, thus mitigating the risk of encountering unexpected token errors when parsing their JSON data.
Fix 3: Eliminating Comma-Related Issues
Comma-related issues are a frequent source of JSON parse error unexpected tokens, as JSON parsers expect very specific comma usage. Here’s how to solve these:
- Step 1: Check Comma After Elements: Ensure that each element in an array or each key-value pair in an object has a comma following it if it’s not the last element or pair. Missing commas can confuse the parser, as it expects a delimiter between elements or pairs.
- Step 2: Remove Trailing Commas: Conversely, having a trailing comma after the last element or pair can also result in an error. JSON does not permit trailing commas like some programming languages do. Carefully inspect the end of lists and objects and remove any unnecessary commas.
- Step 3: Use a Structured Editor: An editor with structured data support, like a JSON-specific tool, can automatically manage commas for you. It’s a worthwhile investment to use tools that help reduce these simple, yet easy-to-overlook mistakes.
- Step 4: Review Nested Structures: Nested structures can get particularly complex. Verify that commas are correctly placed at each level of your nested arrays or objects. Each nested level must adhere to the same comma rules as the top level.
- Step 5: Test in Segments: If your JSON document is large, test it in segments to isolate the section with the comma issue. This can be easier than trying to debug a very large file all at once.
Fix 4: Encoding and Special Characters
Problems with file encoding or special characters can throw JSON parsers off. Here’s what to do:
- Step 1: Remove Byte Order Mark (BOM): Some text editors insert a BOM at the beginning of UTF-8 files. This can cause a JSON parser to fail. Use a tool that can remove the BOM or save the file without it.
- Step 2: Escape Special Characters: All special characters within strings must be escaped. This includes quotes (\”), backslashes (\\), and control characters like newlines (\n) and carriage returns (\r).
- Step 3: Replace Smart Quotes: Smart quotes or curly quotes, often found in text edited in word processors, are invalid in JSON. Replace any smart quotes with straight double quotes.
- Step 4: Handle Multiline Strings: JSON does not support multiline strings. If your data includes line breaks, you need to handle them appropriately by using escape characters or by concatenating strings for each new line.
- Step 5: Validate Against a Schema: If you have a JSON schema, validate your JSON against it. A schema can specify the required structure, the necessary type of each element, and even the specific format of strings.
By meticulously addressing comma placement and ensuring the correct handling of encoding and special characters, you can prevent many of the common JSON parsing issues. The key is always attention to detail—JSON requires precision, and giving it the attention it deserves will save time and frustration.
Fix 5: Correcting Unescaped Characters
Unescaped characters within strings can wreak havoc on JSON parsing, leading to the dreaded JSON parse error unexpected token. Here’s the proper way to address these sneaky culprits:
- Step 1: Identify Unescaped Characters: Go through your JSON strings and look for characters that may need escaping. Common examples include backslashes (\), forward slashes (/), double quotes within strings, and control characters like newlines (\n) and tabs (\t).
- Step 2: Escape Appropriately: Once identified, escape these characters by prefixing them with a backslash (\). For instance, a newline should appear as \\n in a JSON string, and a double quote as \”.
- Step 3: Use String Literals: In many programming environments, you can use string literals that handle escaping for you. This can be a safer way to ensure that your JSON strings are formatted correctly.
- Step 4: Automate with Tools: Consider using a tool or library function that converts your strings to valid JSON strings, handling the escaping as needed. This can prevent manual errors.
- Step 5: Review and Test: Review your changes and test the JSON data to ensure that all strings are correctly escaped and that no unexpected tokens are thrown by the parser.
Fix 6: Addressing BOM and File Corruption Issues
A Byte Order Mark (BOM) or a corrupted file might be invisible when opening a JSON file in a text editor, but they can cause a JSON parser to stumble, causing unexpected token errors. Here’s how to rectify these issues:
- Step 1: Detect BOM Presence: Use a hex editor or a tool capable of showing hidden characters to check for a BOM at the beginning of your file. The BOM is a sequence of bytes (EF BB BF) that signals the encoding of the text file and can disrupt JSON parsing.
- Step 2: Remove BOM: If a BOM is present, save the file without it. Many text editors and IDEs have settings to save files as ‘UTF-8 without BOM’.
- Step 3: Check for Corruption: Look for signs of file corruption, which may include strange characters, irregular formatting, or failure to open in different text editors. If you suspect file corruption, you may need to restore the JSON data from a backup.
- Step 4: Validate File Integrity: Use checksums or hash values to validate the integrity of the file if you have a reference checksum or hash to compare it with. This can help ensure the file has not been corrupted or altered.
- Step 5: Prevent Future Corruption: To prevent future file corruption, ensure that you’re using reliable storage and backup systems. Additionally, use version control systems like Git to manage changes to your JSON files.
By escaping all special characters and ensuring that the file is free from BOMs and corruption, you will eliminate a large swath of common issues that lead to JSON parse error unexpected token. Regular checks and the use of appropriate tools can keep your JSON data in top form, parse-ready for your applications.
Fix 7: Resolving Issues with Comments in JSON
One aspect of JSON that can lead to an unexpected token error is the attempt to include comments. JSON does not support comments within the data structure, and any attempt to include them can cause parsing to fail. Let’s look at how to tackle this:
- Step 1: Remove Comments: If you have comments in your JSON file, remove them. JSON is data-only, and comments are not a part of the data structure. While some parsers may allow comments as an extension of the JSON standard, they are not universally supported.
- Step 2: Externalize Documentation: Instead of comments within the JSON, document your structure and fields externally. Use a README file or documentation within the code that processes the JSON to explain the structure and any nuances that might otherwise be commented directly in the JSON.
- Step 3: Use a Development Environment: Leverage the features of a development environment that flags comments as errors within JSON files. This will help you avoid mistakenly leaving comments in your JSON data.
Fix 8: Parsing JSON with the Correct Method or Library
Sometimes, the method or library you’re using to parse JSON may not be suitable for your specific use case or data format, leading to unexpected token errors. Here’s how to address this:
- Step 1: Understand Your JSON Source: If you’re receiving JSON data from an external source, make sure you understand its structure and encoding. This can affect how you should parse it.
- Step 2: Choose the Right Parsing Method: Select a parsing method or library that is robust and suitable for your data. For example, in JavaScript, JSON.parse() is commonly used, but if you’re dealing with JSONP (JSON with Padding) or a similar structure, you’ll need a different approach.
- Step 3: Handle Edge Cases: Your JSON might include edge cases such as very large numbers or deeply nested objects. Ensure the parser you’re using can handle these cases without throwing an error.
- Step 4: Update Your Libraries: Outdated libraries or methods can be less forgiving when parsing JSON. Ensure you’re using the latest version of whatever library or method you’re employing.
- Step 5: Test with Different Parsers: If you’re encountering issues, try parsing the JSON with a different method or library to see if the problem persists. This can help isolate whether the issue is with the JSON data or the parser itself.
- Step 6: Parse in a Controlled Environment: Before deploying your JSON parsing strategy into a live environment, test it in a controlled setting with varied JSON samples. This can help catch unexpected token errors that might not show up in simpler tests.
By ensuring that comments are not included in the JSON and by selecting and properly utilizing the appropriate parsing method, you can avoid many common pitfalls that result in JSON parse error unexpected token. These fixes aim to maintain the integrity of the JSON data and the effectiveness of the parsing process.
Conclusion
Troubleshooting a JSON parse error unexpected token is a common part of a developer’s life. By understanding the possible reasons for the error and how to methodically fix it, you can save time and avoid frustration. Remember, prevention is better than cure. Adopting robust coding practices, validating your JSON, and being cautious with external data are your best defenses against these parsing pitfalls.
Ultimately, maintaining a keen eye for detail and a disciplined approach to coding and data handling will keep the unexpected token error at bay and ensure your applications run smoothly, parsing JSON data with the precision and efficiency that it’s known for.
FAQs
This error typically pops up when there’s a typo, incorrect structure, or non-compliant syntax in your JSON file.
Start by checking for missing or extra commas, correct data types, and ensure strings are enclosed in double quotes.
No, comments can break JSON structure, as the format doesn’t support them.
Absolutely, JSON expects commas between array items and object members, except after the last element.
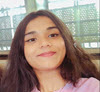
Prachi Mishra is a talented Digital Marketer and Technical Content Writer with a passion for creating impactful content and optimizing it for online platforms. With a strong background in marketing and a deep understanding of SEO and digital marketing strategies, Prachi has helped several businesses increase their online visibility and drive more traffic to their websites.
As a technical content writer, Prachi has extensive experience in creating engaging and informative content for a range of industries, including technology, finance, healthcare, and more. Her ability to simplify complex concepts and present them in a clear and concise manner has made her a valuable asset to her clients.
Prachi is a self-motivated and goal-oriented professional who is committed to delivering high-quality work that exceeds her clients’ expectations. She has a keen eye for detail and is always willing to go the extra mile to ensure that her work is accurate, informative, and engaging.