Non-Arithmetic Combinational Circuits
Non-Arithmetic Combinational Circuits: Circuits blocks are one of the pillars of digital electronics. Whether it be the function of data routing, waveform generation, display of characters, or memory layout, these circuits are enduring everywhere.
In the previous article (links are at the end of the article), we have discussed courses that are capable of performing arithmetic operations. Another category available is the Non-Arithmetic Combinational Circuits. However, their functions revolve around logically encoding and decoding the data provided. Here in this write-up, we will be discussing all the famous combinational circuits.
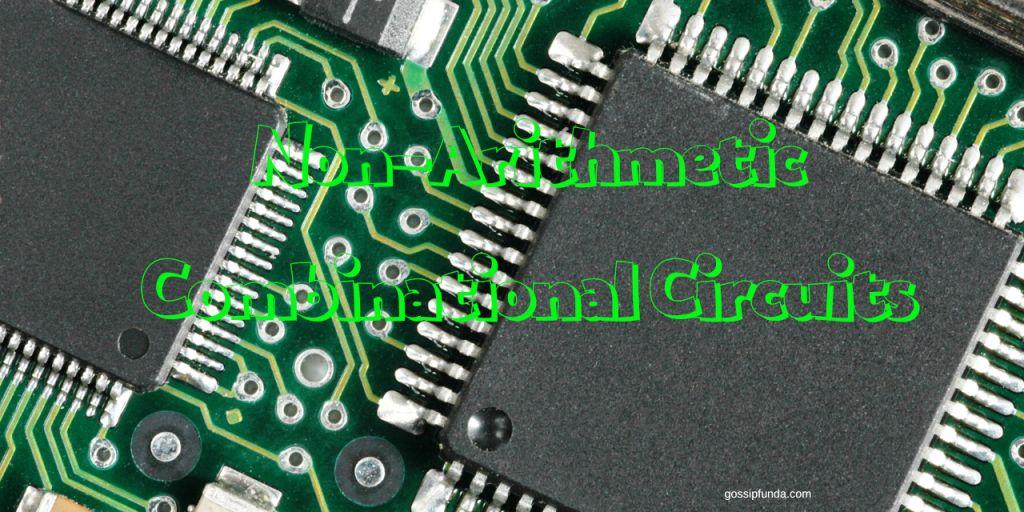
Multiplexer
The Multiplexer is a unique, versatile, and one of the most widely used standard circuits in the digital subject. It is a combinational circuit where one of the data input is routed to the output based on the select signals. Additionally, a set of selection lines controls the selection of a particular input line. Generally, for the most part, there are ‘2^n’ input lines and ‘n’ determination lines whose bit mixes figure out which info is chosen .
The followings are synonym of a Multiplexer
- Data selector
- Many to one circuit
- Parallel to serial converter
- Universal logic converter
- Waveform generator
2 to 1 Mux
The figure shows the logic circuitry for 2:1 MUX. It has two input lines as I0 and I1 and one data select line S. The output line is designated as Y.
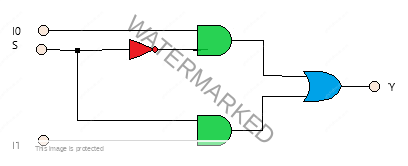
In order to model the code below, we used data flow modeling. The essence of the system lies with the logical operator. So, if data select is high, input port b transfers data to output. However, when data select is low, port a pipelines data to c.
module mux2_1(c,a,b,s);
output c;
input a,b,s;
assign c= s ? b : a ;
endmodule
Here, the design style is gate-level modeling. Therefore through the arrangement, one can understand the entire circuit design.
module mux2_1(c,a,b,s);
output c;
input a,b,s;
wire x1,x2,s1;
not (s1,s);
and a1 ( x1,a,s1);
and a2 (x2, b,s);
or (c,x1,x2);
endmodule

4 to 1 Mux
Code for 4:1 line multiplexer is below. We apply the four outputs I0,I1,I2, and I3 at the input port of MUX and logic level to the selection lines S0 and S1. The code depicts the concept of instantiation. We can realize a 4 to 1 mux using three 2 to 1 mux. Therefore, instantiating the 2 to 1 mux thrice in the construct will serve the purpose.
module mux4_1(y,x1,x2,x3,x4,z1,z2);
output y;
input x1,x2,x3,x4,z1,z2;
wire w1,w2;
mux2_1 m1 (w1,x1,x2,z1);
mux2_1 m2 (w2,x3,x4,z1);
mux2_1 m3 (y,w1,w2,z2);
endmodule
module mux2_1(c,a,b,s);
output c;
input a,b,s;
wire x1,x2;
assign s1=~s;
and a1 ( x1,a,s1);
and a2 (x2, b,s);
or (c,x1,x2);
endmodule
module mux4_1tb;
wire y;
reg x1,x2,x3,x4,z1,z2;
mux4_1 dut(y,x1,x2,x3,x4,z1,z2);
initial
begin
$monitor(y,x1,x2,x3,x4,z1,z2);
$dumpfile("dump.vcd");
$dumpvars;
end
initial
begin
x1=0;
x2=0;
x3=1;
x4=1;
z1=0;
z2=1;
#50
x1=0;
x2=1;
x3=0;
x4=1;
z1=0;
z2=0;
#50
x1=1;
x2=0;
x3=1;
x4=0;
z1=1;
z2=0;
#50
x1=1;
x2=1;
x3=0;
x4=0;
z1=1;
z2=1;
end
endmodule
16 to 1 Mux
A 16 to 1 mux has 16 inputs, 4 data select lines, and 1 output. To actualize any circuit or digital block, there are numerous prospects available. Certainly, we can achieve the respective circuit by pure behavioral style. Another method is by instantiating 4 to 1 mux. Furthermore, 2 to 1 mux will be instantiated to make a 4 to 1 mux course. The code below follows the sheer behavioral model.
We have a 16 bit input a, 4 bit select line s and an output b. We can consider 16-bit input as an array storing 16 elements. 4-bit select can have a sequence from 0 to 15. Hence, the vital expression in the code infers that 4-bit select can act as an index to pick an element from the array and bypass it to the output.
module mux16_1(b,a,s);
output b;
input [15:0] a;
input [3:0] s;
assign b= a[s];
endmodule
module mux16_1tb;
wire b;
reg [15:0] a;
reg [3:0] s;
mux16_1 dut(b,a,s);
initial
begin
$monitor(b,a,s);
$dumpfile("dump.vcd");
$dumpvars;
end
initial
begin
a=16'h3f0a;
s=4'h0;
#5
s=4'h1;
#5
s=4'hc;
end
endmodule
Demultiplexer
A demultiplexer is the reverse of Multiplexer. It is a combinational circuit that gets data on a solitary line and transmits this on one of the ‘2^n’ conceivable yield lines. However, the bit values of ‘n’ selection lines control the selection of a specific output line.
A ‘decoder’ with an enable input can function as a ‘demultiplexer.’ A demultiplexer is also known as:
- Data distributor
- Serial to parallel converter
- One to many circuits
Moreover, similar to Multiplexer, demux has many varients, like 1 to 2, 1 to 4, 1 to 16, etc. The below code models a 1 to 8 demultiplexer. It has 1 input, 3 data select lines, and 8 outputs.
1 to 8 Demux
Below is the code of 1-8 demux. There are 1 input, 8 outputs, and 3 data selection lines. Although, the the modeling style here is data flow.
module Demultiplexer(in,s0,s1,s2,d0,d1,d2,d3,d4,d5,d6,d7);
input in,s0,s1,s2;
output d0,d1,d2,d3,d4,d5,d6,d7;
assign d0=(in & ~s2 & ~s1 &~s0),
d1=(in & ~s2 & ~s1 &s0),
d2=(in & ~s2 & s1 &~s0),
d3=(in & ~s2 & s1 &s0),
d4=(in & s2 & ~s1 &~s0),
d5=(in & s2 & ~s1 &s0),
d6=(in & s2 & s1 &~s0),
d7=(in & s2 & s1 &s0);
endmodule
module TestModule;
reg in;
reg s0;
reg s1;
reg s2;
wire d0;
wire d1;
wire d2;
wire d3;
wire d4;
wire d5;
wire d6;
wire d7;
Demultiplexer uut (
.in(in),
.s0(s0),
.s1(s1),
.s2(s2),
.d0(d0),
.d1(d1),
.d2(d2),
.d3(d3),
.d4(d4),
.d5(d5),
.d6(d6),
.d7(d7)
);
initial begin
$monitor(in,s0,s1,s2,d0,d1,d2,d3,d4,d5,d6,d7);
$dumpfile("dump.vcd");
$dumpvars;
end
initial begin
in = 0;
s0 = 1;
s1 = 1;
s2 = 0;
#100;
in = 1;
s0 = 0;
s1 = 1;
s2 = 0;
#100;
end
endmodule

Encoder
An encoder is an non- arithmatic combinational circuit that performs the reverse of a decoder. It gangs ‘2^n’ input lines, and ‘n’ yield lines. Out of 2^n info lines, only one is activated at a given time and produces an n bit output code, depending upon which input is activated. So like decoder, the encoder also does not have any selection lines.
Encoder convert other codes to binary such as,
- (8 x 3 line) Octal to binary encoder
- (10 x 4 line) Decimal to BCD encoder
- (16 x 4 line) Hexadecimal to binary encoder
8 x 3 Encoder
Octal to binary or 8-3 encoder has 8 inputs and 3 outputs. Unlike multiplexer, it does not have data selection lines.
module Encoder(d0,d1,d2,d3,d4,d5,d6,d7,a,b,c);
input d0,d1,d2,d3,d4,d5,d6,d7;
output a,b,c;
or(a,d4,d5,d6,d7);
or(b,d2,d3,d6,d7);
or(c,d1,d3,d5,d7);
endmodule
module TestModule;
reg d0;
reg d1;
reg d2;
reg d3;
reg d4;
reg d5;
reg d6;
reg d7;
wire a;
wire b;
wire c;
Encoder uut (
.d0(d0),
.d1(d1),
.d2(d2),
.d3(d3),
.d4(d4),
.d5(d5),
.d6(d6),
.d7(d7),
.a(a),
.b(b),
.c(c)
);
initial begin
$monitor(d0,d1,d2,d3,d4,d5,d6,d7,a,b,c);
$dumpfile("dump.vcd");
$dumpvars;
end
initial begin
// Initialize Inputs
d0 = 0;
d1 = 0;
d2 = 0;
d3 = 0;
d4 = 0;
d5 = 0;
d6 = 0;
d7 = 0;
#100;
d0 = 0;
d1 = 0;
d2 = 0;
d3 = 1;
d4 = 0;
d5 = 0;
d6 = 0;
d7 = 0;
// Wait 100 ns for global reset to finish
#100;
end
endmodule

Decoder
A decoder has many inputs and many outputs. Indeed, it is a combinational logic circuit that converts binary information from ‘n’ bit input lines to a maximum ‘2^n’ unique output lines specified only 1 output line activates for every one among possible combinations of input.
Decoders help to identify or convert a particular code, for example:
- Binary to Octal (3-8 line decoders)
- Binary to Hexadecimal (4-16 line decoders)
- BCD to decimal (4-10 line decoders)
- BCD to 7-segment display
However, if the n-bit decoded data has remaining or don’t care combinations, the decoder output will have less than the generalized ‘2^n’ outputs.The memory system of computers widely uses decoders.
2-4 Decoder
A decoder has 2 information sources and 4 yields . Like encoder, it doesn’t have a data selection line.
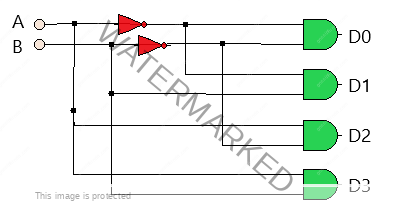
module Decoder(a,b,d0,d1,d2,d3);
input a,b;
output d0,d1,d2,d3;
assign d0=(~a&~b),
d1=(~a&b),
d2=(a&~b),
d3=(a&b);
endmodule
module TestModule;
reg a;
reg b;
wire d0;
wire d1;
wire d2;
wire d3;
Decoder uut (
.a(a),
.b(b),
.d0(d0),
.d1(d1),
.d2(d2),
.d3(d3)
);
initial begin
$monitor(a,b,d0,d1,d2,d3);
$dumpfile("dump.vcd");
$dumpvars;
end
initial begin
a = 0;
b = 0;
#100;
a = 1;
b = 0;
#100;
a=1;
b=1;
end
endmodule

Hazards
Hazard is a phenomenon that may cause the digital circuit to malfunction. These are undesirable transients that may show up at the yield of a course in light of the fact that various ways display diverse propogation delays. Such a transient is also called a ‘glitch’ or a ‘false spike,’ which is caused by the dangerous behavior of the logic circuit.
Hazards occur in Non-Arithmetic Combinational Circuits as well as sequential circuits. In combinational circuits, they may cause a temporary bogus output, though, in sequencial circuits, they may bring about a progress to a wrong stable state. It is, therefore, necessary to check for possible hazards, determine whether they can cause an improper operation to ensure no disturbance at the end. Hazards are classified as:
- Static hazard
- Dynamic hazard
- Essential hazard
Static Hazard
Static hazard occurs in combinational circuits and hence, can be eliminated by using redundant gates. We can divide the static hazard into:
- Static -1
- Static-0
If the input goes from one to zero, the output must remain at logic one, but somehow it momentarily goes to zero. It depicts Static-1 hazard. This hazard happens in 2-level AND – OR implementation. Moreover, if the output remains at zero and instantly goes to one, it is a static-0 hazard. It occurs in 2-level OR – AND implementation.
Dynamic Hazard
When the output changes several times then it should change from ’10’ or ’01’ only once, it is called dynamic hazard.Dynamic hazard occurs when the output changes for two adjacent input combinations while switching, the output should change only once; but it may change three or more times in short intervals because of different delays in several paths. Dynamic hazards occur only in the multilevel circuit.
Essential Hazard
An essential hazard is another type of hazard that occurs in the asynchronous sequential circuit. It is because of inconsistent delays along at least two ways, that began from a similar information. An inordinate delay through an inverter circuit in contrast with the one related with the feedback way may cause such a peril. Basic danger can’t right by including redundant gates as in static hazards. However, the problem solves by adjusting the amount of delay in the affected path.
My previous blog links are here, Don’t miss
Modeling Basic Gates through Verilog
Modeling of Universal and Special Gates on Verilog
Designing Combinational Circuits through Verilog HDL
Modeling of Combinational Logic Circuits
Conclusion
In this very article, we saw the Non-Arithmetic Combinational Circuits. Moreover, we modeled each of them using Verilog hardware description language. Additionally, we discussed the hazards that should be kept in mind while designing any circuits. In upcoming texts, we will discuss Sequential Circuits.

I am an electronics and communication graduate. I qualified GATE in the same domain in 2019. Due to the appetite for VLSI, I am going to join the Hong Kong University of Science and Technology for Masters in IC design this year. My interest in writing evoked during college time when I wrote technical essays for college fest. Apart from it, I am a part of a few local NGOs too.
Such a nice read! Amazing work!